In the world of computer science, Data Structures and Algorithms are two fundamental concepts that shape the way software is developed. From creating efficient applications to solving complex problems, understanding these building blocks is essential for every programmer.
Data structures are the means by which we store and organize data, while algorithms define the steps we take to process and manipulate that data. Together, they form the backbone of software development, ensuring that programs run optimally and efficiently.
In this article, we will explore the importance of data structures and algorithms, delve into common types of data structures, discuss essential algorithms, and understand why mastering these concepts is vital for any programmer.
What Are Data Structures?
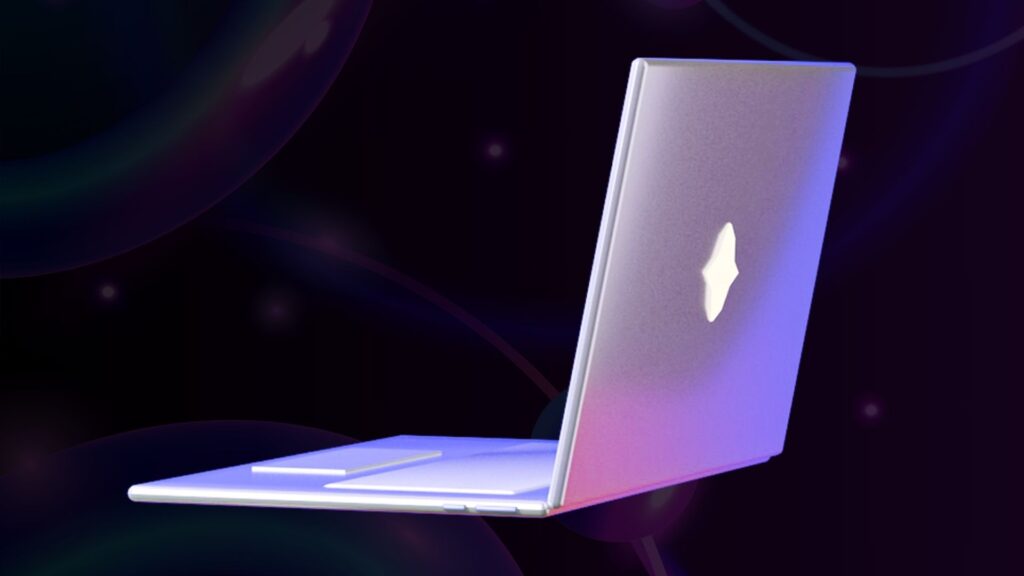
A data structure is a method of organizing and storing data in a way that allows for efficient access and modification. Data structures are the building blocks of programs because they directly influence the efficiency of various operations, such as searching, inserting, updating, and deleting data.
There are two main categories of data structures:
1. Primitive Data Structures
These are the most basic types of data structures. They include:
- Integers: Whole numbers used for calculations.
- Floats: Decimal numbers for representing real values.
- Characters: Single letters or symbols.
- Booleans: True/false values.
These primitive types are the foundation for more complex data structures.
2. Non-Primitive Data Structures
These structures are built using primitive data types and can store multiple values. The most common non-primitive data structures are:
- Arrays: A collection of elements stored in a contiguous block of memory, where each element is of the same data type.
- Linked Lists: A linear data structure where elements (nodes) are connected using pointers, making it easier to insert or delete elements.
- Stacks: A collection that follows the Last In First Out (LIFO) principle, where the most recently added element is the first to be removed.
- Queues: A collection that follows the First In First Out (FIFO) principle, where the first element added is the first one to be removed.
- Trees: Hierarchical structures consisting of nodes connected by edges. The most common type is the binary tree, where each node has at most two children.
- Graphs: Used to represent networks, such as social media connections or transport systems, where nodes are connected by edges.
What Are Algorithms?
An algorithm is a step-by-step procedure or set of rules used to solve a specific problem or perform a computation. In simple terms, an algorithm is a recipe for solving a problem.
Algorithms are critical in software development because they directly affect the performance of applications. An inefficient algorithm can lead to slow, resource-draining programs, while a well-designed algorithm can significantly optimize performance.
There are several types of algorithms, but some of the most commonly used include:
- Sorting Algorithms: These algorithms arrange data in a specific order, such as ascending or descending. Popular sorting algorithms include Bubble Sort, Merge Sort, and Quick Sort.
- Search Algorithms: These algorithms help in searching for a specific element within a data structure. Some common search algorithms are Linear Search and Binary Search.
- Graph Algorithms: These algorithms solve problems related to graph data structures, such as finding the shortest path between nodes or determining if a graph is connected. Examples include Dijkstra’s Algorithm and Depth First Search (DFS).
- Dynamic Programming: This approach solves problems by breaking them down into simpler subproblems and storing the results of these subproblems to avoid redundant work. Examples include the Fibonacci Sequence and the Knapsack Problem.
The Importance of Data Structures and Algorithms
Understanding data structures and algorithms is crucial for several reasons:
- Efficiency: A well-chosen data structure can significantly improve the performance of an application. For example, using a hash table instead of an array for lookups can reduce the time complexity of a search operation from O(n) to O(1).
- Optimization: Many real-world problems involve large datasets. Efficient algorithms and data structures are necessary to handle this data in a time-efficient and memory-efficient manner.
- Problem Solving: Data structures and algorithms are powerful tools for solving complex problems. From artificial intelligence to system design, they form the basis of many advanced topics in computer science.
- Interview Preparation: If you’re pursuing a career in software development, interviews often test your knowledge of data structures and algorithms. Mastering these concepts will make you more competitive in the job market.
Common Data Structures and Their Applications
Now that we’ve covered the basics, let’s look at a few common data structures and their real-world applications.
1. Arrays
Arrays are one of the simplest and most commonly used data structures. They are ideal when you need to store a fixed-size collection of elements and access them by index. Arrays are used in everything from simple data storage to complex algorithms like Dynamic Programming.
Example Application: Storing student grades in an online learning platform.
2. Linked Lists
Linked lists are great for dynamic memory allocation. Unlike arrays, they allow you to insert and remove elements more easily. However, they have higher memory overhead due to the pointers that link each node.
Example Application: Managing data in a playlist or an undo/redo operation in software.
3. Stacks
Stacks follow the LIFO (Last In, First Out) principle. They are commonly used in algorithms that involve recursion, such as Depth First Search (DFS).
Example Application: Undo functionality in software or tracking function calls in a program.
4. Queues
Queues follow the FIFO (First In, First Out) principle. They are often used in scheduling tasks and managing resources in systems like printers or servers.
Example Application: Print job management in an office setting.
5. Trees
Trees are used in situations where hierarchical relationships need to be represented. Binary trees, for instance, are often used in databases for quick lookups.
Example Application: Organizing file directories in an operating system.
6. Graphs
Graphs are essential when relationships between various entities need to be represented. They are used extensively in social networks, route planning, and recommendation systems.
Example Application: Facebook friend connections or Google Maps route navigation.
Understanding Time and Space Complexity
When choosing algorithms and data structures, it’s important to consider time complexity and space complexity. These measure how the efficiency of an algorithm or data structure scales with increasing input sizes.
- Time Complexity: This refers to the amount of time an algorithm takes to complete as the input grows. Common time complexities include O(1) (constant time), O(n) (linear time), O(log n) (logarithmic time), and O(n^2) (quadratic time).
- Space Complexity: This refers to the amount of memory an algorithm uses relative to the input size. Efficient algorithms use less memory, making them suitable for resource-constrained environments.
Conclusion
Data structures and algorithms are at the core of computer science and programming. Whether you’re developing a simple app or a complex system, having a solid understanding of these concepts is vital for writing efficient, scalable, and maintainable code.
Mastering data structures and algorithms will not only help you solve problems effectively but also enhance your programming skills and prepare you for technical interviews. Remember, the key is practice. Keep experimenting with different data structures, solving algorithmic challenges, and refining your understanding — and you’ll be well on your way to becoming a proficient software developer.