Title: Unlocking the Power of Java: A Comprehensive Guide for Beginners and Beyond
Java has stood the test of time as one of the most popular and versatile programming languages in the world. As it continues to be a top choice for developers worldwide. In this article, we will take an in-depth look at Java programming, exploring its history, core concepts, common uses, and how you can get started as a Java developer.
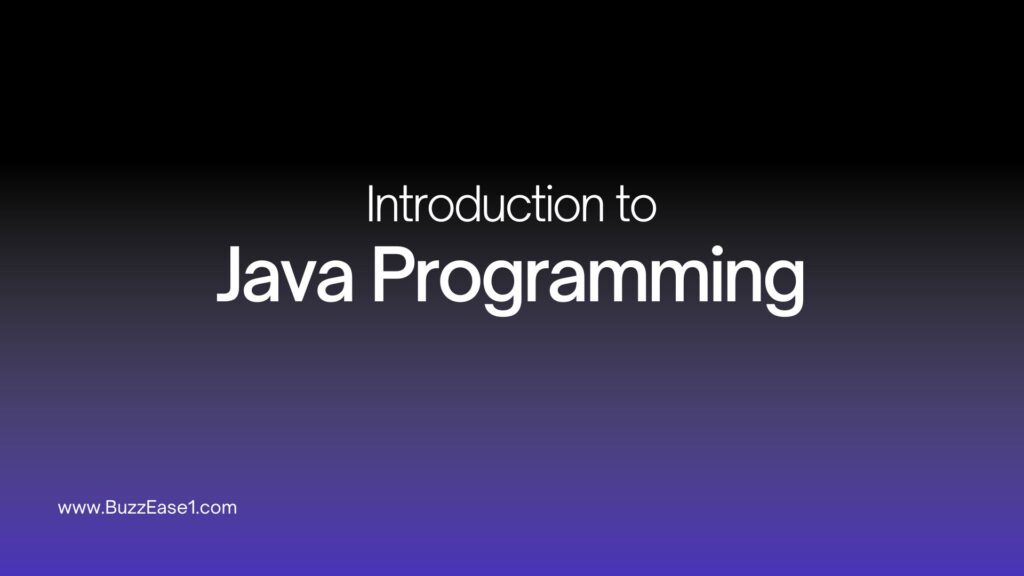
The Birth and Evolution of Java
Java was initially developed by Sun Microsystems in 1991, under the name “Oak”. The goal was to create a language that could run on various devices, from microwaves to set-top boxes, and still deliver robust performance. Over the years, Java evolved into the language we know today, thanks to its “Write Once, Run Anywhere” (WORA) philosophy, which allows Java applications to run on any system that has a Java Virtual Machine (JVM).
In 2009, Oracle Corporation acquired Sun Microsystems, and since then, Oracle has continued to maintain and evolve Java. The language is now widely used in diverse sectors, from mobile applications (Android) to cloud computing and even big data analytics.
Why Choose Java?
When it comes to choosing a programming language, there are plenty of options out there. So, why should Java be your go-to choice? let you show some reasons:
- Platform Independence: Java’s hallmark is its platform independence. With the JVM, developers can write code once and run it on any platform that supports Java. This capability makes Java a favorite for cross-platform applications.
- Large Community and Ecosystem: Java has a massive developer community that contributes to an ever-growing ecosystem of frameworks, libraries, and tools. This support makes it easier to solve problems and build complex applications efficiently.
- Rich API: Java comes with a comprehensive set of standard libraries and APIs that cater to almost any need, whether you’re building graphical user interfaces (GUIs), working with databases, or handling networking.
- Object-Oriented: Java is an object-oriented programming (OOP) language, which helps in organizing code into reusable structures. This leads to cleaner, more maintainable code and easier debugging and testing.
- Performance: Interpreted languages like Python can be slower than compiled languages, Java strikes a good balance. The JVM optimizes bytecode, offering competitive performance for many types of applications.
- Security: Java’s security features make it a great choice for developing secure applications. Because the JVM’s sandbox model ensures that potentially harmful code doesn’t affect the host system, and features like bytecode verification help catch security flaws before runtime.
Core Concepts of Java Programming
Before you dive into coding with Java, it’s important to understand the core concepts that form the foundation of the language.
1. Classes and Objects
At the heart of Java programming is the concept of classes and objects. A class is a blueprint for creating objects, which are instances of that class. Objects can contain data in the form of fields (also called attributes or properties) and methods to manipulate that data.
Example:
class Car {
String model;
int year;
void start() {
System.out.println("The car is starting.");
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car(); // Create an object of the Car class
myCar.model = "Toyota";
myCar.year = 2020;
myCar.start();
}
}
2. Inheritance
Inheritance allows a new class to inherit properties and behaviors (fields and methods) from an existing class. This is a key feature of object-oriented programming that promotes code reuse and helps create a hierarchical structure.
Example:
class Animal {
void sound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Bark");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.sound(); // Output: Bark
}
}
3. Polymorphism
Polymorphism allows you to call the same method on different objects, with each object responding in its own way. This makes code more flexible and easier to extend.
Example:
class Animal {
void sound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Bark");
}
}
class Cat extends Animal {
void sound() {
System.out.println("Meow");
}
}
public class Main {
public static void main(String[] args) {
Animal animal1 = new Dog();
Animal animal2 = new Cat();
animal1.sound(); // Output: Bark
animal2.sound(); // Output: Meow
}
}
4. Encapsulation
Encapsulation is the concept of hiding the internal state of an object and only exposing a controlled interface. This is typically done by declaring fields as private and providing public getter and setter methods.
Example:
class Person {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person();
person.setName("John");
System.out.println(person.getName());
}
}
5. Abstraction
Abstraction allows you to hide complex implementation details and expose only the essential features of an object. In Java, abstraction can be achieved through abstract classes and interfaces.
Example:
abstract class Animal {
abstract void sound();
}
class Dog extends Animal {
void sound() {
System.out.println("Bark");
}
}
public class Main {
public static void main(String[] args) {
Animal dog = new Dog();
dog.sound(); // Output: Bark
}
}
Common Uses of Java
Java’s versatility makes it suitable for a variety of applications, including:
- Web Development: Developers often use Java in server-side application, and frameworks like Spring and Java Server Faces (JSF) help developers build robust, scalable web applications.
- Mobile Development: Java is the official language for Android development. With the Android SDK, developers can create mobile applications for millions of devices.
- Enterprise Solutions: Java is the language of choice for large enterprise solutions. Tools like Java EE (Enterprise Edition) and Spring provide frameworks for building scalable and secure business applications.
- Big Data: Big data frameworks like Hadoop widely use Java., which helps process and analyze massive datasets across distributed computing environments.
- Game Development: java is not as popular as other languages like C++ But Java is still used in game development, especially for Android-based mobile games.
- Scientific Applications: Java’s portability and stability make it suitable for scientific applications that require complex algorithms and calculations.
Starting with Java
If you’re eager to start coding in Java, here’s a step-by-step guide to get you going:
- Install Java: First, download and install the Java Development Kit (JDK) from the official Oracle website or adopt OpenJDK for an open-source alternative.
- Set Up an IDE: While you can write Java code in any text editor, using an Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or NetBeans can make coding easier. These IDEs provide features like syntax highlighting, code completion, and debugging tools.
- Write Your First Program: After installing Java and setting up your IDE, create a simple program, like the “Hello, World!” example below, to make sure everything is working correctly.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
- Understand the Syntax: Familiarize yourself with Java’s syntax, including how to define variables, write loops, use conditionals, and create methods.
- Practice: The key to mastering Java is consistent practice. Start by writing small programs and gradually build up to more complex projects.
Conclusion
Java continues to be an essential tool for developers due to its versatility, reliability, and strong community support. By understanding Java’s core concepts, exploring its common uses, and practicing regularly, you’ll be well on your way to becoming proficient in Java programming. Whether you’re building web applications, mobile apps, or enterprise solutions, Java offers the foundation you need to succeed in today’s tech-driven world.